In this article, we are going to look into,
- what is grpc-gateway
- what is the use of it and what problem does it solves
- steps to create gRPC proxy gateway to serve the REST clients.
- some examples
grpc-gateway
The gRPC-Gateway is a plugin of the Google protocol buffers compiler protoc. It reads protobuf service definitions and generates a reverse-proxy server which translates a RESTful HTTP API into gRPC. This server is generated according to the
google.api.http
annotations in your service definitions.This helps you provide your APIs in both gRPC and RESTful style at the same time. — grpc-gateway doc
problems solved by grpc-gateway
gRPC is great — it generates API clients and server stubs in many programming languages, it is fast, easy-to-use, bandwidth-efficient and its design is combat-proven by Google.
However, you might still want to provide a traditional RESTful API as well. Reasons can range from maintaining backwards-compatibility, supporting languages or clients not well supported by gRPC to simply maintaining the aesthetics and tooling involved with a RESTful architecture.
Simply,
- to support the existing REST clients
- Public API expectations is HTTP/JSON
use of grpc-gateway
gRPC-Gateway provides the capability to serve both the REST and gRPC clients with the little extra annotations in the proto file.
We define all our service and rpcs in our proto file as usual, to support the REST clients we need to make some annotations to the RPCs and compile the proto file with the gRPC-Gateway generator.(need to install the plugin)
It acts as the reverse proxy that translates the gRPC to REST and vice versa.
grpc-gateway project aims to provide that HTTP+JSON interface to your gRPC service. A small amount of configuration in your service to attach HTTP semantics is all that’s needed to generate a reverse-proxy with this library. — grpc-gateway doc
It also provides Swagger/OpenAPI generator.
Steps to create gRPC proxy gateway to serve the REST clients
- Install neccessary plugins for protoc
protoc-gen-go to generate pb.go file
protoc-gen-go-grpc to generate grpc.pb.go file
protoc-gen-grpc-gateway to generate pb.gw.go file
- Annotate the RPCs in the proto file (REST definition) by importing the “google/api/annotations.proto” file.
- Compile the proto file by all the 3 plugins becoz we need 3 files at the end.
$ protoc -I<path/to/protofile> --go_out=<outdir> --go-grpc_out=<outdir> --grpc-gateway_out=<outdir> protofilename.proto
- Configure the gRPC server to serve both the gRPC and REST clients (both in the different sockets). The config is just a few lines. Serving REST Clients with the help of proxy grpc-gateway. This proxy grpc-gateway connects to the gRPC server as the gRPC client and serves the REST Clients.
grpc-gateway in action
Protoc plugins
- protoc go plugin
- protoc go grpc plugin
- grpc-gateway plugin.
$ go install google.golang.org/protobuf/cmd/protoc-gen-go@v1.28
$ go install google.golang.org/grpc/cmd/protoc-gen-go-grpc@v1.2
$ go install github.com/grpc-ecosystem/grpc-gateway/v2/protoc-gen-grpc-gateway@v2.10.3
// v2.10.3 is the latest one at the time of writing this article
This installs the bin file in the $GOPATH/bin
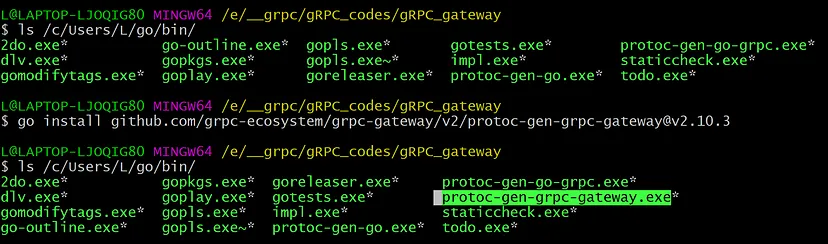
create proto file
Have created one sample proto file in my $GOPATH/src/github.com/myUsername/projectname/
syntax = "proto3"
option go_package = "github.com/favtuts/grpc_gateway/proto/sample";
message MessageOne {
string id = 1;
string msg = 2;
}
message MessageID {
string id = 1;
}
service MessageService {
rpc getMessage(MessageID) returns (MessageOne);
}
This proto file is the regular one which is used to serve the grpc clients. To make it to serve REST clients we need to do some annotations.
Now we have to import the google/api/annotations.proto to our proto file. And made some annotation.
Unfortunately while importing the google/api/annotations.proto we get error “was not found”. Yes the error is correct, the annotations.proto is not present in our Projects dir.

We have to manually include them in our Project folder.

Now the error is gone after we have the google folder under our project root dir.
import "google/api/annotations.proto"